mc¶
![digraph mc {
initial [label="Initial configuration\n\nCalculate Energy E", shape=box];
change [label="Change a variable at random", shape=box];
calc [label="Calculate ΔE", shape=box];
dE [label="ΔE ?", shape=diamond];
initial -> change -> calc -> dE;
keep [label="Keep new\nconfiguration", shape=box];
keep2 [label="Keep new\nconfiguration\nwith probability P", shape=box];
dE -> keep [label="ΔE < 0"];
dE -> keep2 [label="ΔE > 0"];
repeat [label="Repeat until E reaches minimum", shape=box];
keep -> repeat;
keep2 -> repeat;
repeat -> change;
}](../_images/graphviz-bbded4aba3e2c42f89130590075d45fcfc4dba07.png)
where
(Source code, png, hires.png, pdf)
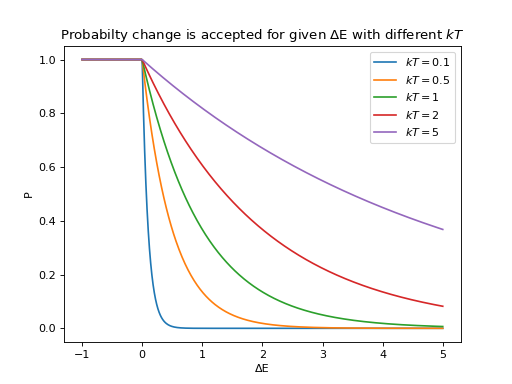
-
class
javelin.mc.
MC
[source]¶ MonteCarlo class
For the Monte Carlo simulations to run you need to provide an input structure, target (neighbor and energy set) and modifier. A basic, do nothing, example is shown:
>>> from javelin.structure import Structure >>> from javelin.modifier import BaseModifier >>> from javelin.energies import Energy >>> structure = Structure(symbols=['Na','Cl'],positions=[[0,0,0],[0.5,0.5,0.5]]) >>> >>> energy = Energy() >>> neighbors = structure.get_neighbors() >>> >>> mc = MC() >>> mc.add_modifier(BaseModifier(0)) >>> mc.temperature = 1 >>> mc.cycles = 2 >>> mc.add_target(neighbors, energy) >>> >>> new_structure = mc.run(structure) <BLANKLINE> Cycle = 0, temperature = 1.0 Accepted 0 good, 1 neutral (dE=0) and 0 bad out of 1 <BLANKLINE> Cycle = 1, temperature = 1.0 Accepted 0 good, 1 neutral (dE=0) and 0 bad out of 1 >>>
-
add_target
(neighbors, energy)[source]¶ This will add an energy calculation and neighbour pair that will be used to calculate and energy for each modification step. You can add as many targets as you like.
Parameters: - neighbour (
javelin.neighborlist.NeighborList
or n x 5 array of neighbor vectors) – neighbour for which the energy will be calculated over - energy (
javelin.energies.Energy
) – the energy function that will be calculated for each neighbor
- neighbour (
-
cycles
¶ The number of cycles to perform.
-
iterations
¶ The number of iterations (site modifications) to perform for each cycle. Default is equal to the number of unitcells in the structure.
-
modifier
¶ This is how the structure is to be modified, must be of type
javelin.modifier.BaseModifier
.
-
run
(structure, inplace=False)[source]¶ Execute the Monte Carlo routine. You must provide the structure to modify as a parameter. This will by default this will return a new
javelin.structure.Structure
with the results, to modify the provided structure in place set inplace=TrueParameters: structure ( javelin.structure.Structure
) – structure to run the Monte Carlo on
-
temperature
¶ Temperature parameter (
) which changes the probability (P) a energy change of
is accepted
Temperature can be a single value for all cycles or you can provide a different temperature for each cycle. This allows you to do quenching of the disorder. If you provide more temperatures than cycles then only the first temperatures corresponding to the number of cycles are used. If there are more cycles than temperature than for remaining cycles the last temperature in the list will be used.
>>> mc = MC() >>> mc.temperature = 0.1 >>> print(mc) Number of cycles = 100 Temperature[s] = 0.1 Structure modfifiers are [] >>> >>> mc.temperature = np.linspace(1, 0, 6) >>> print(mc) Number of cycles = 100 Temperature[s] = [ 1. 0.8 0.6 0.4 0.2 0. ] Structure modfifiers are []
-